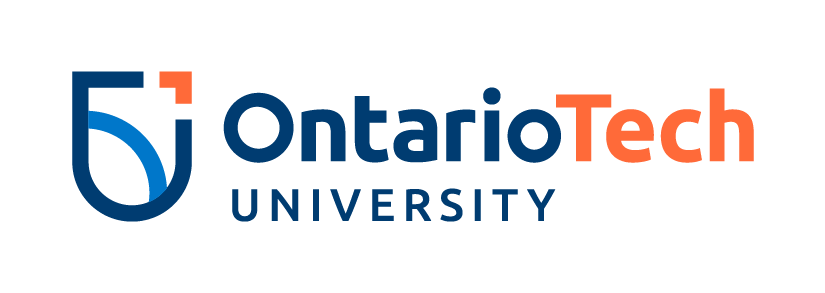
Monte Carlo integration¶
Faisal Qureshi
faisal.qureshi@ontariotechu.ca
http://www.vclab.ca
Copyright information¶
© Faisal Qureshi
License¶

In [1]:
import random
import numpy as np
import matplotlib.pyplot as plt
In [2]:
def monte_carlo_integration(func, num_samples):
total = 0
for _ in range(num_samples):
x = random.random()
total += func(x)
average = total / num_samples
return average
In [3]:
def f(x):
return x**2
In [4]:
plt.figure(figsize=(5,5))
x_all = np.linspace(-2,2,1024)
y_all = f(x_all)
x = np.linspace(0,1,256)
y = f(x)
plt.plot(x_all,y_all,'r')
plt.fill_between(x,y)
Out[4]:
<matplotlib.collections.FillBetweenPolyCollection at 0x10aa697f0>
In [5]:
estimated_integral = monte_carlo_integration(f, num_samples=100)
exact_integral = 1/3 # Exact value of the integral
print("Estimated Integral of f:", estimated_integral)
print("Exact Integral of f:", exact_integral)
Estimated Integral of f: 0.3200380458458901 Exact Integral of f: 0.3333333333333333
In [ ]: