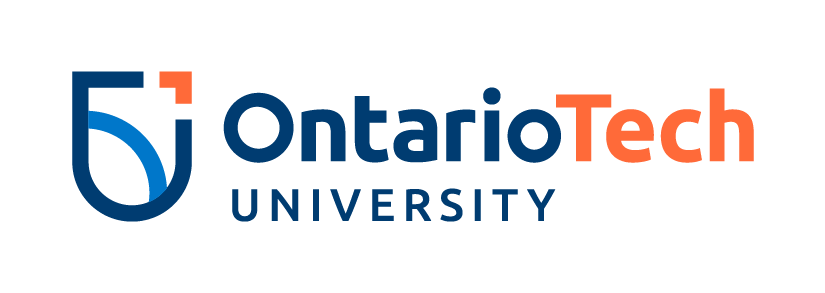
Linear Congruential Method¶
Generating Uniform Random Numbers
Faisal Z. Qureshi
faisal.qureshi@ontariotechu.ca
Copyright information¶
© Faisal Qureshi
License¶

In [1]:
import numpy as np
import matplotlib.pyplot as plt
LCM Method¶
Generates the next number given the current number x and values for m, a, and c.
In [2]:
def lcm(x,a,c,m):
return (a * x + c) % m
Generates n numbers starting from x
In [3]:
def gen(n,x0,a,c,m):
lastx = x0
l = [lastx]
for i in range(1,n):
newx = lcm(lastx,a,c,m)
l.append(newx)
lastx = newx
x = np.array(l)
return x
The shuffle technique¶
In [4]:
def shuffle(n, x0, a, c, m):
x = np.zeros(n)
k = 100000
v = gen(k+1,x0,a,c,m)
lastx = v[-1]
v = v / float(m)
for i in range(0,n-1):
newx = lcm(lastx, a, c, m)
j = int(newx * k / float(m))
x[i] = v[j]
lastx = newx
newx = lcm(lastx, a, c, m)
v[j] = newx / float(m)
lastx = newx
return x
Visualizations¶
In [5]:
def histogram(x, n, bins, fignum, a, c, m, s):
hist, bins = np.histogram(x,bins = bins)
width = 0.7* (bins[1]-bins[0])
center = (bins[:-1]+bins[1:])/2
plt.figure(fignum, figsize=(3,3))
plt.title("Histogram (%s): a=%d, c=%d, m=%d" % (s, a, c, m) )
plt.bar(center, hist, align = 'center', width = width)
Bad values for a, c, m¶
In [6]:
n = 1000000
x0 = 0
a = 200
c = 1000
m = 65000
x = gen(n,x0,a,c,m) / float(m)
histogram(x, n, 50, 1, a, c, m, 'LCM')
In [7]:
n = 1000000
x0 = 0
a = 4023
c = 200000
m = 800000
x = gen(n,x0,a,c,m) / float(m)
histogram(x, n, 50, 3, a, c, m, 'LCM')
Good values for a, c, and m¶
In [9]:
n = 1000000
x0 = 0
a = 4095
#a = 4096
c = 150889
m = 714025
x = gen(n,x0,a,c,m) / float(m)
histogram(x, n, 50, 2, a, c, m, 'LCM')
Using shuffle method¶
In [21]:
n = 1000000
x0 = 0
a = 4096
c = 150889
m = 714025
x = shuffle(n,x0,a,c,m)
histogram(x, n, 50, 4, a, c, m, 'Shuffle')
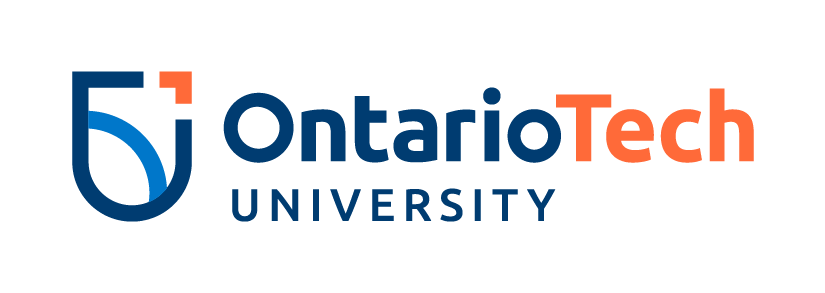
In [ ]: