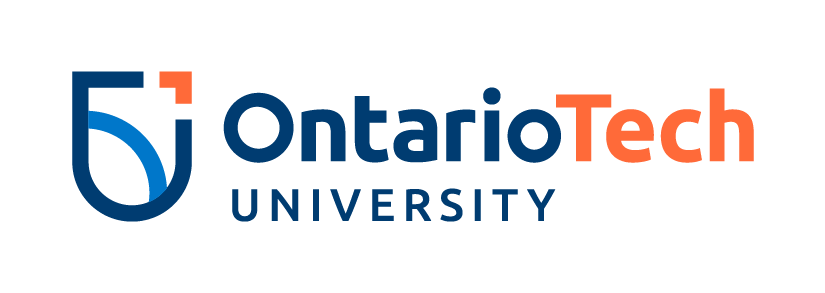
Generating Random Numbers from Exponential distribution¶
Faisal Qureshi
faisal.qureshi@ontariotechu.ca
Copyright information¶
© Faisal Qureshi
License¶

In [1]:
import numpy as np
import matplotlib.pyplot as plt
Using numpy's method¶
In [2]:
n = 100000
x = np.empty(n)
for i in range(n):
x[i] = np.random.exponential()
plt.figure(figsize=(4,4))
plt.title(f'Exponential')
plt.hist(x, bins=np.linspace(0,10,100));
Using cdf inverse method¶
In [3]:
def inv_cdf_of_exponential(mu, u):
return - mu * np.log( u )
def gen_samples_from_exponential_using_inv_cdf(n):
mu = 1
x = np.empty(n)
for i in range(0,n):
u = np.random.rand()
x[i] = inv_cdf_of_exponential(mu, u)
return x
Sample histogram¶
In [4]:
x_cdf = gen_samples_from_exponential_using_inv_cdf(n)
plt.figure(figsize=(4,4))
plt.title(f'Exponential')
plt.hist(x, bins=np.linspace(0,10,100), color='blue', alpha=0.2, label='builtin');
plt.hist(x_cdf, bins=np.linspace(0,10,100), color='red', alpha=0.2, label='inv cdf');
plt.legend()
Out[4]:
<matplotlib.legend.Legend at 0x11419a7b0>
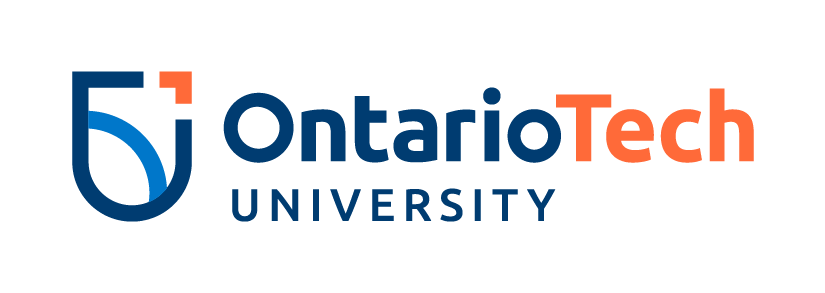
In [ ]: