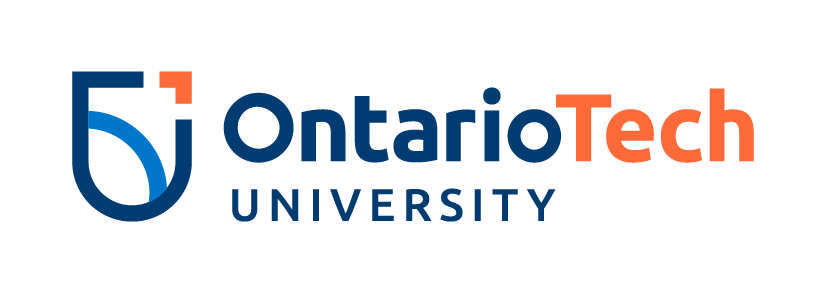
Yield
Keyword in Python¶
Faisal Qureshi
faisal.qureshi@ontariotechu.ca
http://www.vclab.ca
Copyright information¶
© Faisal Qureshi
License¶

Yield
Keyword¶
- The
yield
keyword is used in the context of generator functions. - A generator function is a special type of function that allows you to generate a series of values lazily, on-the-fly, as they are needed, instead of generating them all at once and storing them in memory.
- When a generator function is called, it returns an iterator object, which can be iterated over using a loop or by using functions like
next()
. - The
yield
keyword is what makes a function a generator function. Whenyield
is used inside a function. - The
yield
pauses the function's execution and returns a value to the caller. The next time the function is called, it picks up from where it left off, remembering its previous state.
Generate numbers method¶
In [2]:
def generate_numbers_1(a, b):
result = []
for i in range(a, b):
result.append(i)
return result
In [3]:
numbers = generate_numbers_1(30,35)
print(numbers)
[30, 31, 32, 33, 34]
In [4]:
for i in generate_numbers_1(30, 35):
print(i,end=' ')
30 31 32 33 34
In [17]:
for i in generate_numbers_1(10, 20):
print(i, end=' ')
10 11 12 13 14 15 16 17 18 19
Generate numbers method (using yield
keyword)¶
In [7]:
def generate_numbers_2(a, b):
for i in range(a, b):
yield i
In [8]:
print(generate_numbers_2(30,35))
<generator object generate_numbers_2 at 0x107ca3e60>
In [9]:
for i in generate_numbers_2(10, 20):
print(i, end=' ')
10 11 12 13 14 15 16 17 18 19
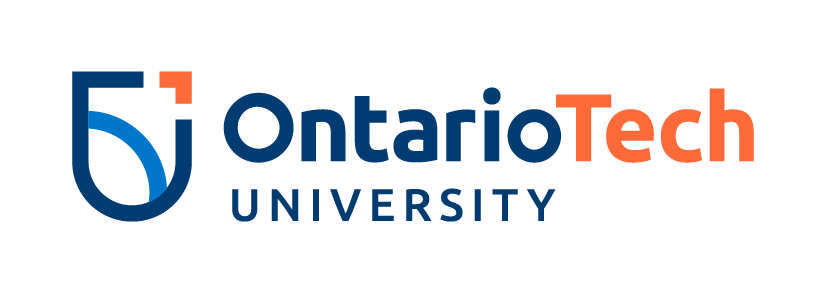