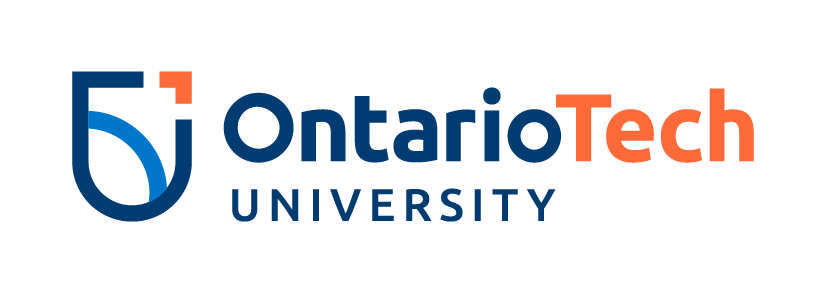
Modeling a Car Wash¶
Faisal Qureshi
faisal.qureshi@ontariotechu.ca
http://www.vclab.ca
Copyright information¶
© Faisal Qureshi
License¶

Setup¶
A carwash shop has a limited number of washing stations. Each car takes "some time" to be washed. The time that each car takes is bounded by some range, e.g., it may take a car to be washed any where between 3 and 7 minutes. Each washing station can only clean a single are at each time. Cars arrive at the carwash shop, wait for a wash station to become available, and gets washed.
Acknowledgements¶
This code is adapted from simpy
examples.
In [1]:
import random
import simpy
In [7]:
class Carwash(object):
def __init__(self, env, num_wash_stations, washtimes):
self.env = env
self.wash_stations = simpy.Resource(env, num_wash_stations)
self.washtimes = washtimes
def wash(self, car):
a, b = washtimes
washtime = random.randint(a, b)
print(washtime)
yield self.env.timeout(washtime)
In [8]:
def car(env, name, cw):
print(f'{name} arrives at the carwash shop at {env.now}')
with cw.wash_stations.request() as request:
yield request
print(f'{name} enters the carwash at {env.now}')
yield env.process(cw.wash(name))
print(f'{name} leaves the carwash at {env.now}')
In [9]:
def setup(env,
num_wash_stations,
wash_times,
time_between_arrivals):
carwash_shop = Carwash(env, num_wash_stations, time_between_arrivals)
i = 0
while True:
a, b = time_between_arrivals
arrival_time = random.randint(a, b)
yield env.timeout( arrival_time )
i += 1
env.process( car(env, 'car %d' % i, carwash_shop) )
In [10]:
print('Carwash Simulation')
seed = 42
random.seed(seed)
num_wash_stations = 2
washtimes = (5,8)
time_between_arrivals = (3,6)
simulation_duration = 20
env = simpy.Environment()
env.process( setup(env, num_wash_stations, washtimes, time_between_arrivals) )
env.run(until=simulation_duration)
Carwash Simulation car 1 arrives at the carwash shop at 3 car 1 enters the carwash at 3 7 car 2 arrives at the carwash shop at 6 car 2 enters the carwash at 6 6 car 3 arrives at the carwash shop at 10 car 1 leaves the carwash at 10 car 3 enters the carwash at 10 5 car 2 leaves the carwash at 12 car 4 arrives at the carwash shop at 14 car 4 enters the carwash at 14 8 car 3 leaves the carwash at 15 car 5 arrives at the carwash shop at 17 car 5 enters the carwash at 17 5
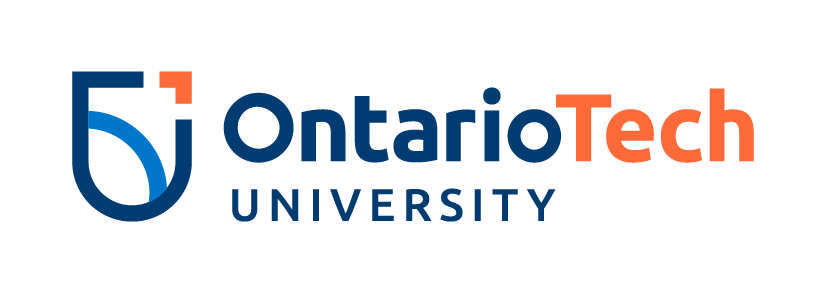
In [ ]: